Web Element Operations
Context:
Once we identify elements using a suitable locator/selector strategy , we will need to interact with the elements. For a text field – set text, for a checkbox – check, select list – select an option and so on.
The api’s/methods we will be using depends on the type of WebElement we interact with. For example, we can sendKeys() to a text field, click() on a link etc. In ths section, we will interact with a web form having different kinds of html elements (text box, radio, checkbox, select etc.). Some of the common web element operations are as follows:
- sendKeys()
- click()
- selectByVisibleText()
- selectByValue()
- getAttribute()
- …Many more
Pre-requisites:
- You have completed the section “Set up Env” and have your environment ready with JDK, maven and Eclipse (+plugins)
- You have read and understood HTML DOM
- You were able to comprehend “Find Element Strategies” post
- You downloaded the project code base for class3 under – https://github.com/machzqcq/CucumberJVMExamples and imported into Eclipse and the structure should look as below
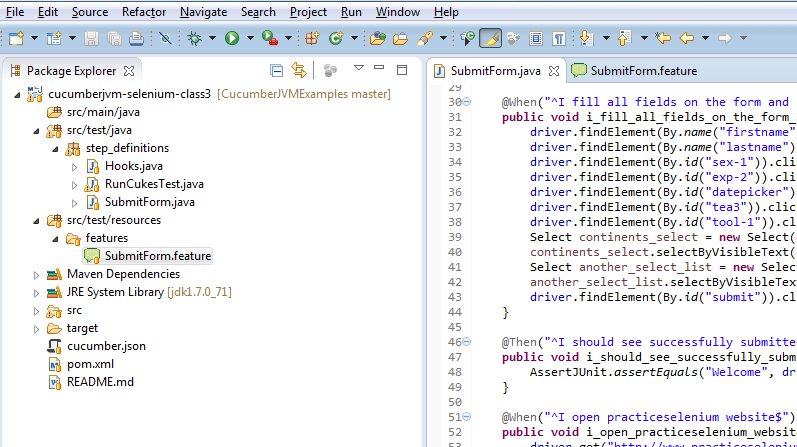
If you are using intellij [which I would recommend over Eclipse], then imported project should look as below
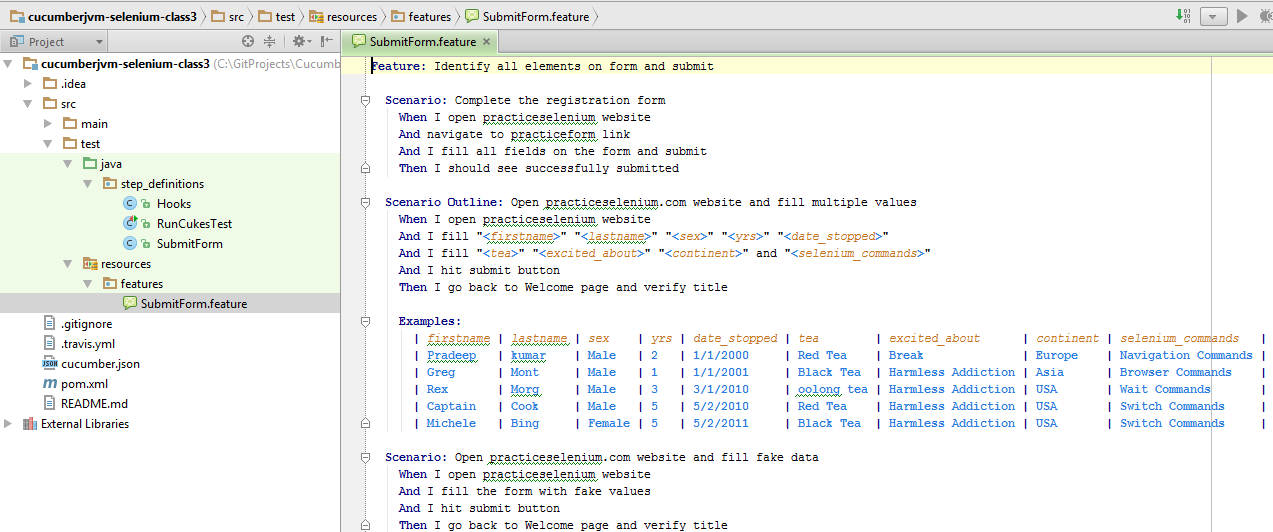
Syntax:
|
driver.findElement(By.name(“firstname”)).sendKeys(“firstname”);
|
|
driver.findElement(By.id(“sex-1”)).click();
|
|
Select another_select_list = new Select(driver.findElement(By.id(“selenium_commands”)));
another_select_list.selectByVisibleText(“Navigation Commands”);
|
|
Select continents_select = new Select(driver.findElement(By.id(“continents”)));
continents_select.selectByVisibleText(continent);
|
- We use sendKeys() method and send any string we want to set inside the text field
- We use click() to click on a link or button or any WebElement
- Select is a type in itself, hence we wrap a WebElement into a Select type and then select its options using selectByVisibleText and pass the option text to it.
Scenario Outline:
If we need to execute a set of cucumber steps with different data sets, we can use scenario outline. We will talk in detail about scenario outline again in “Intermediate Tutorial”, however I thought to include a scenario here, because it is applicable on a form that we will use in this section.
Faker
There will be situations where we might have to key in fake data into fields. In this section we will use a library Faker that will key in random data. In the pom.xml, include the below dependency.
|
<dependency>
<groupId>com.github.javafaker</groupId>
<artifactId>javafaker</artifactId>
<version>0.5</version>
</dependency>
|
Scenario 1
In this scenario, we fill the form on “http://www.practiceselenium.com/practice-form.html” with values and then submit the form. We call the scenario “Complete registration form
|
Scenario: Complete the registration form
When I open practiceselenium website
And navigate to practiceform link
And I fill all fields on the form and submit
Then I should see successfully submitted
|
step_defintions/Submitform.java
|
@When(“^I open practiceselenium website$”)
public void i_open_practiceselenium_website() throws Throwable {
driver.get(“http://www.practiceselenium.com/practice-form.html”);
AssertJUnit.assertEquals(“practice-form”, driver.getTitle());
}
|
|
@When(“^navigate to practiceform link$”)
public void navigate_to_practiceform_link() throws Throwable {
driver.get(“http://www.practiceselenium.com/practice-form.html”);
AssertJUnit.assertEquals(“practice-form”, driver.getTitle());
}
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
@When(“^I fill all fields on the form and submit$”)
public void i_fill_all_fields_on_the_form_and_submit() throws Throwable {
driver.findElement(By.name(“firstname”)).sendKeys(“firstname”);
driver.findElement(By.name(“lastname”)).sendKeys(“lastname”);
driver.findElement(By.id(“sex-1”)).click();
driver.findElement(By.id(“exp-2”)).click();
driver.findElement(By.id(“datepicker”)).sendKeys(“1/1/2000”);
driver.findElement(By.id(“tea3”)).click();
driver.findElement(By.id(“tool-1”)).click();
Select continents_select = new Select(driver.findElement(By.id(“continents”)));
continents_select.selectByVisibleText(“Asia”);
Select another_select_list = new Select(driver.findElement(By.id(“selenium_commands”)));
another_select_list.selectByVisibleText(“Navigation Commands”);
driver.findElement(By.id(“submit”)).click();
}
|
|
@Then(“^I should see successfully submitted$”)
public void i_should_see_successfully_submitted() throws Throwable {
AssertJUnit.assertEquals(“Welcome”, driver.getTitle());
}
|
Scenario 2 (Data Table)
In this scenario, we fill the form on “http://www.practiceselenium.com/practice-form.html” with various data sets supplied as a data table. This is extremely powerful for data-driven framework and we will examine more in Intermediate tutorial.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
Scenario Outline: Open practiceselenium.com website and fill multiple values
When I open practiceselenium website
And I fill “<firstname>” “<lastname>” “<sex>” “<yrs>” “<date_stopped>”
And I fill “<tea>” “<excited_about>” “<continent>” and “<selenium_commands>”
And I hit submit button
Then I go back to Welcome page and verify title
Examples:
| firstname | lastname | sex | yrs | date_stopped | tea | excited_about | continent | selenium_commands |
| Pradeep | kumar | Male | 2 | 1/1/2000 | Red Tea | Break | Europe | Navigation Commands |
| Greg | Mont | Male | 1 | 1/1/2001 | Black Tea | Harmless Addiction | Asia | Browser Commands |
| Rex | Morg | Male | 3 | 3/1/2010 | oolong tea | Harmless Addiction | USA | Wait Commands |
| Captain | Cook | Male | 5 | 5/2/2010 | Red Tea | Harmless Addiction | USA | Switch Commands |
| Michele | Bing | Female | 5 | 5/2/2011 | Black Tea | Harmless Addiction | USA | Switch Commands |
|
step_defintions/Submitform.java
|
@When(“^I fill \”(.*?)\” \”(.*?)\” \”(.*?)\” \”(.*?)\” \”(.*?)\”$”)
public void i_fill(String firstname, String lastname, String sex, String yrs, String dateStopped) throws Throwable {
driver.findElement(By.name(“firstname”)).sendKeys(firstname);
driver.findElement(By.name(“lastname”)).sendKeys(lastname);
driver.findElement(By.id(“sex-1”)).click();
driver.findElement(By.id(“exp-2”)).click();
driver.findElement(By.id(“datepicker”)).sendKeys(dateStopped);
}
|
|
@When(“^I fill \”(.*?)\” \”(.*?)\” \”(.*?)\” and \”(.*?)\”$”)
public void i_fill_and(String tea, String excitedAbout, String continent, String seleniumCommands) throws Throwable {
driver.findElement(By.id(“tea3”)).click();
driver.findElement(By.id(“tool-1”)).click();
Select continents_select = new Select(driver.findElement(By.id(“continents”)));
continents_select.selectByVisibleText(continent);
Select another_select_list = new Select(driver.findElement(By.id(“selenium_commands”)));
another_select_list.selectByVisibleText(seleniumCommands);
}
|
Scenario 3 (Fake data)
In this scenario, we fill the form on “http://www.practiceselenium.com/practice-form.html” with fake data generated by Faker a library that can generate random data.
|
Scenario: Open practiceselenium.com website and fill fake data
When I open practiceselenium website
And I fill the form with fake values
And I hit submit button
Then I go back to Welcome page and verify title
|
step_defintions/Submitform.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
@When(“^I fill the form with fake values$”)
public void i_fill_the_form_with_fake_values() throws Throwable {
driver.findElement(By.name(“firstname”)).sendKeys(faker.name().firstName());
driver.findElement(By.name(“lastname”)).sendKeys(faker.name().firstName());
driver.findElement(By.id(“sex-1”)).click();
driver.findElement(By.id(“exp-2”)).click();
driver.findElement(By.id(“datepicker”)).sendKeys(“1/1/2001”);
driver.findElement(By.id(“tea3”)).click();
driver.findElement(By.id(“tool-1”)).click();
Select continents_select = new Select(driver.findElement(By.id(“continents”)));
continents_select.selectByVisibleText(“Asia”);
Select another_select_list = new Select(driver.findElement(By.id(“selenium_commands”)));
another_select_list.selectByVisibleText(“Navigation Commands”);
}
|
Execution Output
IntelliJ Execution (how to execute is explained in previous post)
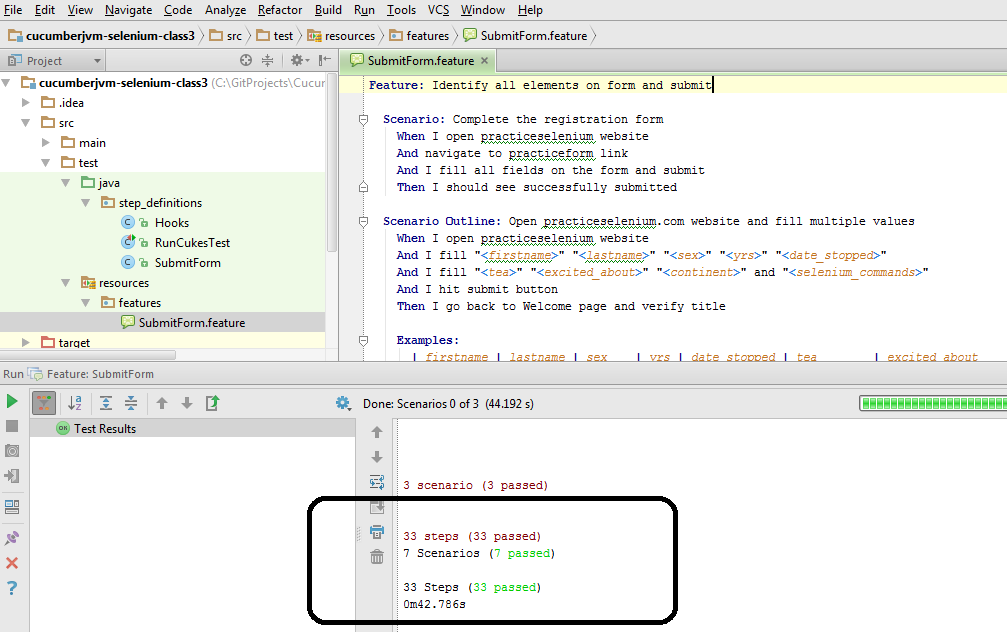
If you executed SubmitForm.feature from eclipse plugin, then it should look similar to the below
Eclipse Execution
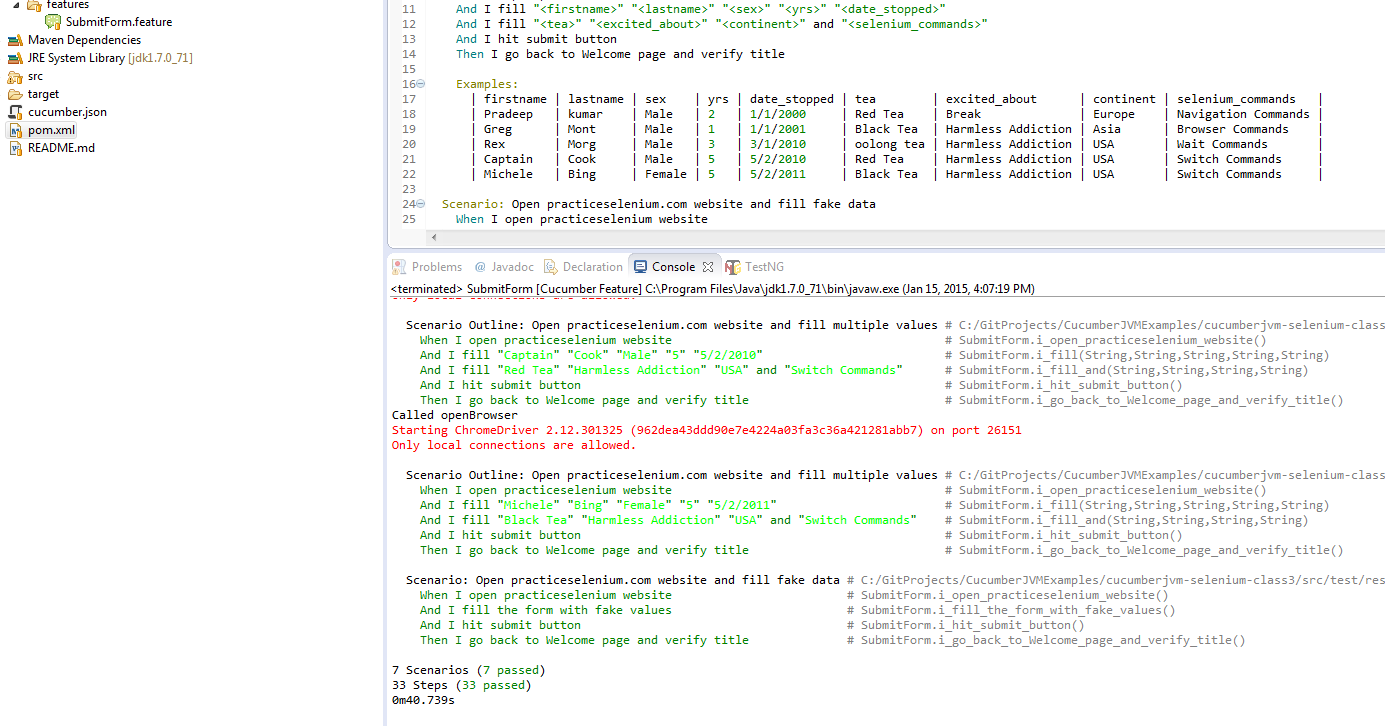
Command Line Execution
If you execute “mvn test” from command line, then the output should be similar to the below
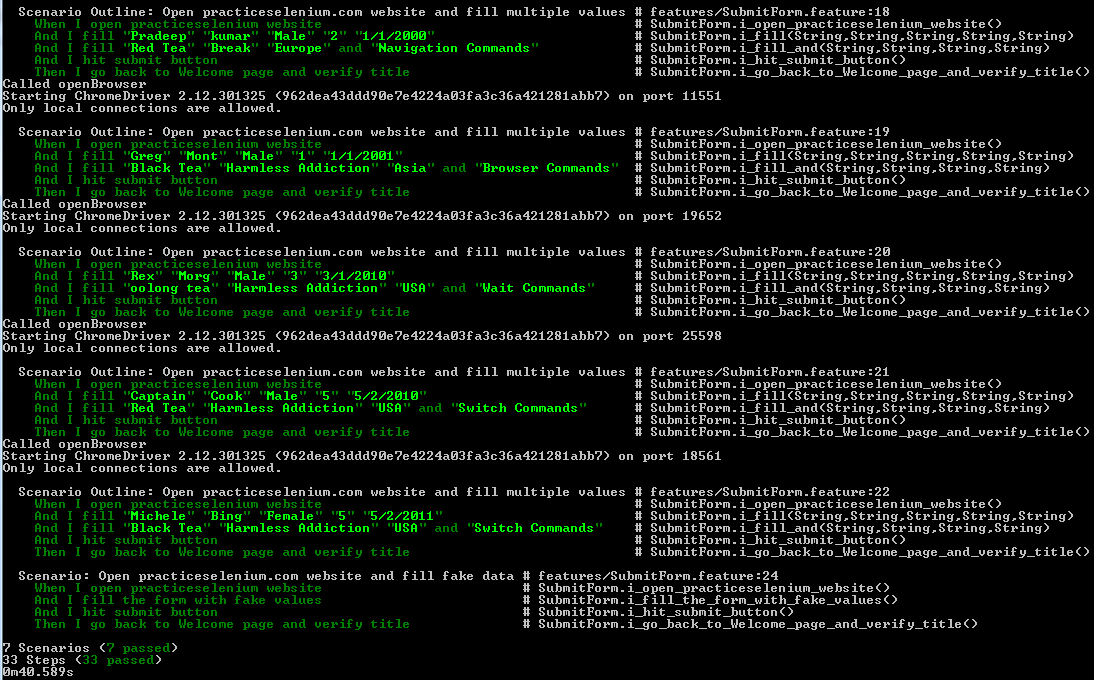
CI Server
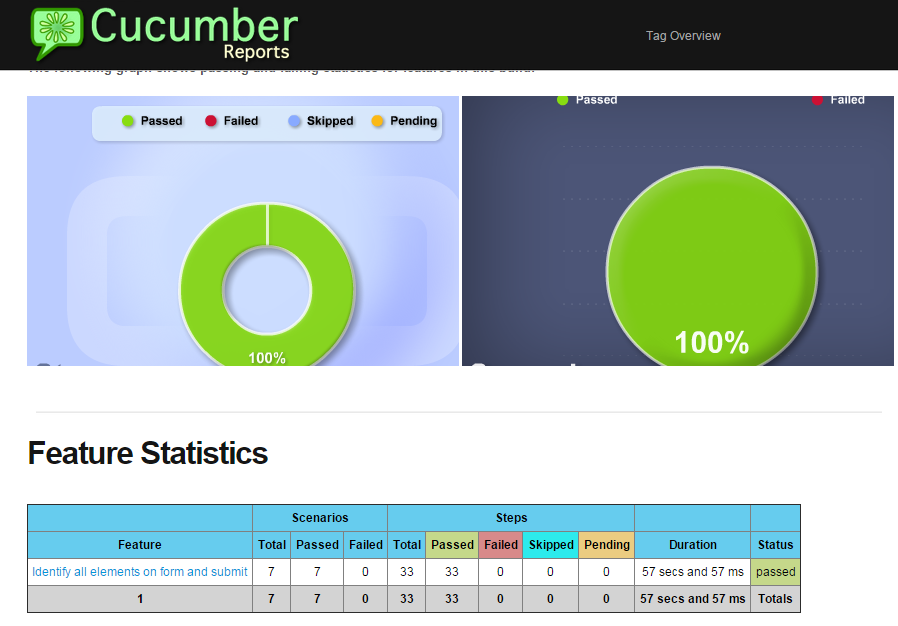
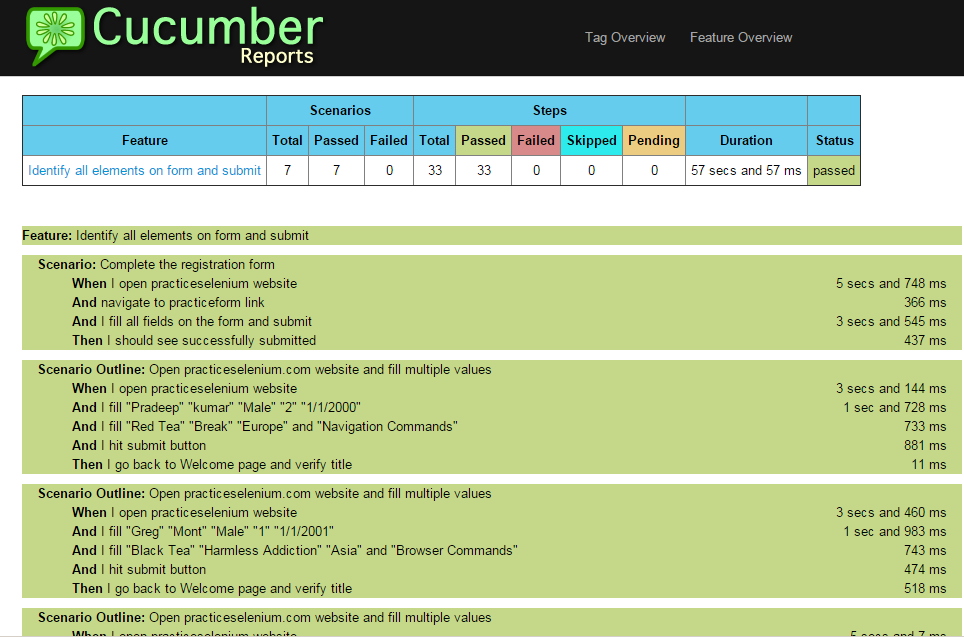